Handling Forms in React Native: Techniques for User Input Validation
In the world of mobile app development, creating forms that efficiently handle user input is a crucial aspect of delivering a seamless user experience. Whether it’s a login screen, a registration form, or a settings panel, users expect their interactions with forms to be smooth and error-free. Achieving this requires not only a solid understanding of React Native, but also the implementation of effective input validation techniques. In this article, we’ll delve into various strategies for handling forms and performing input validation in React Native, accompanied by code samples and best practices.
Forms are the gateway through which users interact with your React Native applications. From logging in to providing personal information, forms are a pivotal point of interaction that can make or break the user experience. While creating aesthetically pleasing forms is essential, ensuring the accuracy and validity of the data users input is equally important. In this article, we’ll explore techniques to handle forms effectively by implementing user input validation in your React Native projects.
1. Setting Up a Basic Form
Before we dive into the intricacies of form validation, let’s set up a basic form in React Native. Assume we’re creating a simple registration form with fields for a user’s name, email, and password.
jsx import React, { useState } from 'react'; import { View, TextInput, Button, StyleSheet } from 'react-native'; const BasicForm = () => { const [name, setName] = useState(''); const [email, setEmail] = useState(''); const [password, setPassword] = useState(''); const handleRegister = () => { // Logic for form submission }; return ( <View style={styles.container}> <TextInput placeholder="Name" value={name} onChangeText={setName} style={styles.input} /> <TextInput placeholder="Email" value={email} onChangeText={setEmail} style={styles.input} /> <TextInput placeholder="Password" value={password} onChangeText={setPassword} secureTextEntry style={styles.input} /> <Button title="Register" onPress={handleRegister} /> </View> ); }; const styles = StyleSheet.create({ container: { padding: 16, }, input: { marginBottom: 12, padding: 10, borderColor: '#ccc', borderWidth: 1, borderRadius: 4, }, }); export default BasicForm;
In this snippet, we’ve created a simple form using the TextInput component for input fields and the Button component for the registration button. The state variables name, email, and password hold the values of the input fields, and the handleRegister function will be responsible for the form submission logic.
2. Understanding Input Validation
Input validation is the process of ensuring that the data entered into a form adheres to specific criteria. This criteria can include mandatory fields, proper data formats, character lengths, and more. Proper input validation serves two primary purposes:
- Data Accuracy: Validating user input prevents incorrect or inconsistent data from being submitted, ensuring that your app’s database remains reliable.
- User Experience: By providing timely feedback and guiding users toward correct input, you enhance the user experience, reducing frustration and errors.
3. Techniques for Input Validation
3.1 Using Built-in HTML5-like Validation Attributes
React Native’s TextInput component provides properties that mimic HTML5 form validation attributes. For example, the required attribute ensures that a field must be filled out before submission, while the pattern attribute enforces a specific format. Here’s how you can use these attributes in your TextInput components:
jsx <TextInput placeholder="Email" value={email} onChangeText={setEmail} style={styles.input} required={true} keyboardType="email-address" /> <TextInput placeholder="Password" value={password} onChangeText={setPassword} secureTextEntry style={styles.input} required={true} minLength={8}
However, relying solely on these attributes might not provide the most user-friendly experience, as the default error messages may not be very informative.
3.2 Implementing Custom Validation Logic
For more control over validation and error messaging, you can implement custom validation functions. Let’s assume we want to ensure that the password contains at least one uppercase letter, one lowercase letter, and one digit. We can define a function like this:
jsx const isStrongPassword = (password) => { const regex = /^(?=.*\d)(?=.*[a-z])(?=.*[A-Z]).{8,}$/; return regex.test(password); };
You can then use this function to validate the password and display a custom error message:
jsx <TextInput placeholder="Password" value={password} onChangeText={setPassword} secureTextEntry style={styles.input} /> {password && !isStrongPassword(password) && ( <Text style={styles.errorText}> Password must contain at least one uppercase letter, one lowercase letter, and one digit. </Text> )}
3.3 Displaying Error Messages Effectively
To provide clear and concise error messages, you can conditionally render error messages below each input field, as shown in the previous example. Styling the error messages with a different color or adding an icon can make them more noticeable. Additionally, consider disabling the submit button if the form contains validation errors to prevent users from submitting incorrect data.
4. Real-time Validation
Real-time validation involves providing instant feedback to users as they type, rather than waiting until they submit the form. This approach enhances the user experience by allowing them to correct errors as they occur.
One way to achieve real-time validation is by using the onChangeText event of the TextInput component to trigger validation functions. For instance, you can immediately check whether the email format is correct and display an error message:
jsx <TextInput placeholder="Email" value={email} onChangeText={(text) => { setEmail(text); setEmailError(!isValidEmailFormat(text)); }} style={styles.input} /> {emailError && ( <Text style={styles.errorText}>Please enter a valid email address.</Text> )}
However, keep in mind that performing validation on every keystroke might lead to performance issues. To mitigate this, consider debouncing the validation function.
5. Form Submission and Feedback
Once the user fills out the form correctly, it’s time to handle the submission process and provide appropriate feedback. Here are a few points to consider:
- Disable the Submit Button: Disable the submit button when the form is invalid to prevent users from attempting to submit incorrect data.
- Success and Error Messages: After submission, provide clear success or error messages to inform the user about the result. For instance:
jsx const handleRegister = () => { if (isValidForm()) { // Perform registration logic setShowSuccessMessage(true); } else { setShowErrorMessage(true); } }; // Within the render function {showSuccessMessage && ( <Text style={styles.successText}>Registration successful!</Text> )} {showErrorMessage && ( <Text style={styles.errorText}>Please fill out all required fields.</Text> )}
6. Library Integration for Enhanced Functionality
While implementing form validation from scratch is a great learning experience, there are libraries that can significantly streamline the process and provide additional features. One popular library is Formik, which offers an efficient way to manage form state, validation, and submission.
To integrate Formik into your project, you can follow these steps:
Install Formik and Yup (a schema validation library) using npm or yarn:
npm install formik yup
or
csharp yarn add formik yup
Import Formik components and Yup validation schemas into your form component:
jsx import { Formik, Form, Field, ErrorMessage } from 'formik'; import * as Yup from 'yup';
Define a validation schema using Yup:
jsx const validationSchema = Yup.object({ name: Yup.string().required('Name is required'), email: Yup.string().email('Invalid email format').required('Email is required'), password: Yup.string() .required('Password is required') .min(8, 'Password must be at least 8 characters long'), });
Wrap your form with the Formik component and provide the validation schema:
jsx <Formik initialValues={{ name: '', email: '', password: '' }} validationSchema={validationSchema} onSubmit={(values) => { // Submit logic }} > {({ errors, touched }) => ( <Form> {/* Form fields and validation messages */} </Form> )} </Formik>
Formik abstracts the management of form state, validation, and submission, making it easier to create complex forms with minimal boilerplate code.
7. Best Practices for Form Validation
As you implement form validation in your React Native apps, keep the following best practices in mind:
- Simplicity: Keep your forms simple and avoid overwhelming users with too many fields.
- Clear and Concise Errors: Use user-friendly error messages that clearly indicate what needs to be corrected.
- Accessibility: Ensure that your forms are accessible to users with disabilities, including proper labeling and error descriptions.
- Testing: Thoroughly test your forms on various devices and screen sizes to ensure a consistent experience.
Conclusion
Handling forms and implementing user input validation are essential skills for building robust and user-friendly React Native applications. By following the techniques and best practices discussed in this article, you’ll be well-equipped to create forms that not only look great but also provide a seamless experience for your users. From basic input validation to advanced library integration, the knowledge you’ve gained will undoubtedly contribute to the success of your app development endeavors. Happy coding!
Table of Contents
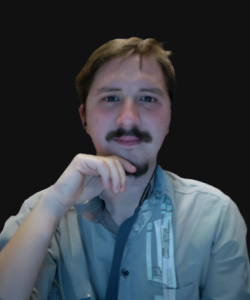
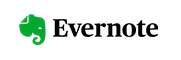