Svelte Form Handling: Creating Interactive and Validated Forms
In the world of web development, forms are the bridge that connects users to the backend services of a website or application. Whether it’s a simple login form or a complex data submission process, creating interactive and validated forms is a crucial aspect of delivering a seamless user experience. Enter Svelte, a modern JavaScript framework that excels in creating efficient and dynamic user interfaces. In this blog post, we’ll explore how Svelte’s form handling capabilities empower developers to build interactive and validated forms with ease.
1. Understanding the Importance of Form Handling
1.1 The Role of Forms in User Interaction
Forms serve as a direct channel for users to input and submit information, making them an integral part of user interaction on websites and applications. From user registrations and logins to feedback submissions, forms facilitate various tasks. An effective form should not only provide a pleasant user experience but also ensure the accuracy and integrity of the data being collected.
1.2 Challenges in Form Handling
Developers often face challenges in creating forms that are both interactive and validated. Handling user input, validating input data, providing real-time feedback, and managing dynamic form elements are tasks that can become complex and time-consuming. This is where Svelte comes to the rescue, offering a streamlined approach to form handling.
2. Getting Started with Svelte Form Handling
2.1 Setting Up a Svelte Project
Before diving into form handling, ensure you have a Svelte project set up. You can create a new Svelte project using the following commands:
bash npx degit sveltejs/template svelte-form-app cd svelte-form-app npm install npm run dev
This sets up a basic Svelte project structure that you can work within.
2.2 Building a Basic Form Component
Let’s start by creating a simple form component. In your Svelte project, navigate to the src folder and create a new file named Form.svelte.
svelte <script> let username = ""; let password = ""; function handleSubmit() { alert("Form submitted!"); } </script> <form on:submit|preventDefault={handleSubmit}> <label> Username: <input type="text" bind:value={username} /> </label> <label> Password: <input type="password" bind:value={password} /> </label> <button type="submit">Submit</button> </form>
In this example, we’ve created a basic form component that captures the username and password input from the user. The handleSubmit function prevents the default form submission behavior and displays an alert. This is just a starting point, but it highlights Svelte’s simplicity in handling forms.
3. Capturing User Input
3.1 Binding Input Fields to Data
Svelte’s two-way data binding simplifies the process of capturing user input. By using the bind:value directive, you establish a connection between the input field and a variable in your script. Any changes made by the user in the input field automatically update the corresponding variable.
svelte <input type="text" bind:value={username} />
3.2 Handling User Input Events
Svelte allows you to listen to various input events and respond to them dynamically. For instance, if you want to perform an action when the user types in the input field, you can use the on:input event.
svelte <input type="text" on:input={event => console.log(event.target.value)} />
3.3 Two-Way Data Binding
Svelte’s two-way data binding not only captures user input but also reflects changes back to the input field. This means that if you update the corresponding variable in your script, the input field’s value will automatically update as well.
svelte <script> let inputValue = "Initial value"; </script> <input type="text" bind:value={inputValue} /> <p>The input value is: {inputValue}</p>
4. Form Validation Made Simple
4.1 Built-in Validation Techniques
Validating user input is crucial for maintaining data integrity. Svelte provides built-in techniques to perform basic validation on form fields. You can use the pattern attribute for input validation and provide a user-friendly error message.
svelte <input type="email" bind:value={email} pattern="[a-z0-9._%+-]+@[a-z0-9.-]+\.[a-z]{2,}$" /> {#if $email.validity.patternMismatch}<p class="error">Invalid email address</p>{/if}
4.2 Custom Validation Functions
For more complex validation scenarios, you can create custom validation functions. These functions can be used to validate input against specific conditions and display appropriate error messages.
svelte <script> let age = ""; function validateAge(input) { const parsedAge = parseInt(input); if (isNaN(parsedAge) || parsedAge < 18) { return "You must be at least 18 years old."; } return ""; } </script> <input type="text" bind:value={age} /> {#if validateAge($age)}<p class="error">{validateAge($age)}</p>{/if}
4.3 Providing User Feedback
Svelte’s reactivity enables real-time feedback to users. By combining validation techniques with conditional rendering, you can instantly show or hide error messages based on user input.
5. Creating Dynamic Form Elements
5.1 Conditional Rendering of Form Fields
Svelte’s conditional rendering makes it easy to show or hide form fields based on certain conditions. This is useful for displaying optional fields only when necessary.
svelte <script> let showAdditionalField = false; </script> <label> <input type="checkbox" bind:checked={showAdditionalField} /> Show additional field </label> {#if $showAdditionalField} <input type="text" placeholder="Additional field" /> {/if}
5.2 Adding and Removing Form Fields Dynamically
In some cases, you might need to allow users to add or remove form fields dynamically. Svelte’s reactive nature simplifies this process.
svelte <script> let dynamicFields = [{ id: 1, value: "" }]; let nextFieldId = 2; function addField() { dynamicFields = [...dynamicFields, { id: nextFieldId, value: "" }]; nextFieldId++; } function removeField(id) { dynamicFields = dynamicFields.filter(field => field.id !== id); } </script> {#each dynamicFields as field (field.id)} <div> <input type="text" bind:value={field.value} /> <button on:click={() => removeField(field.id)}>Remove</button> </div> {/each} <button on:click={addField}>Add Field</button>
5.3 Adapting the Data Model
When dealing with dynamic form elements, your data model needs to adapt to changes. Svelte’s reactivity handles this seamlessly, ensuring that your form state is always in sync with the user interface.
6. Submitting and Processing Data
6.1 Handling Form Submission
Submitting a form in Svelte is straightforward. You can use the on:submit event and a function to handle the form submission logic.
svelte <script> function handleSubmit() { // Form submission logic here console.log("Form submitted!"); } </script> <form on:submit|preventDefault={handleSubmit}> <!-- Form fields here --> <button type="submit">Submit</button> </form>
6.2 Making API Calls
Often, form submissions involve sending data to a backend server. You can use JavaScript’s fetch API or a library like axios to make API calls and handle the response.
svelte <script> import axios from 'axios'; function handleSubmit() { const formData = { // Extract form data here }; axios.post('https://api.example.com/submit', formData) .then(response => { // Handle response }) .catch(error => { // Handle error }); } </script>
6.3 Feedback and Error Handling
Provide clear feedback to users after form submission. Svelte’s reactive nature enables you to display success messages or error messages based on the API response.
Conclusion
Svelte’s form handling capabilities empower developers to create interactive and validated forms efficiently. From capturing user input and implementing validation to managing dynamic form elements and handling data submission, Svelte streamlines the process and ensures a seamless user experience. By leveraging Svelte’s reactivity and simplicity, developers can focus on building engaging forms that meet the needs of modern web applications.
Incorporating Svelte into your form handling workflow opens the door to creating captivating and user-friendly forms that enhance the overall usability of your web applications. Whether you’re building a simple login form or a complex data submission process, Svelte’s intuitive approach to form handling will undoubtedly contribute to a more efficient development process and a delightful user experience.
Table of Contents
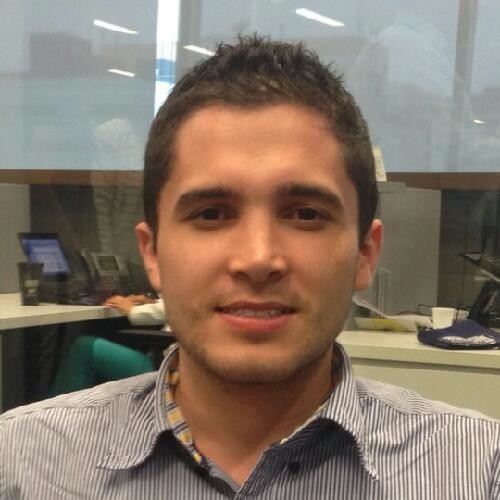
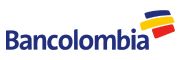