Function prototypes
Function Prototype(Declaration)
- In ‘C’ functions first have to be declared before they are used.
- Prototype lets compiler to know about the return data type, argument list and their data type and order of arguments being passed to the function.
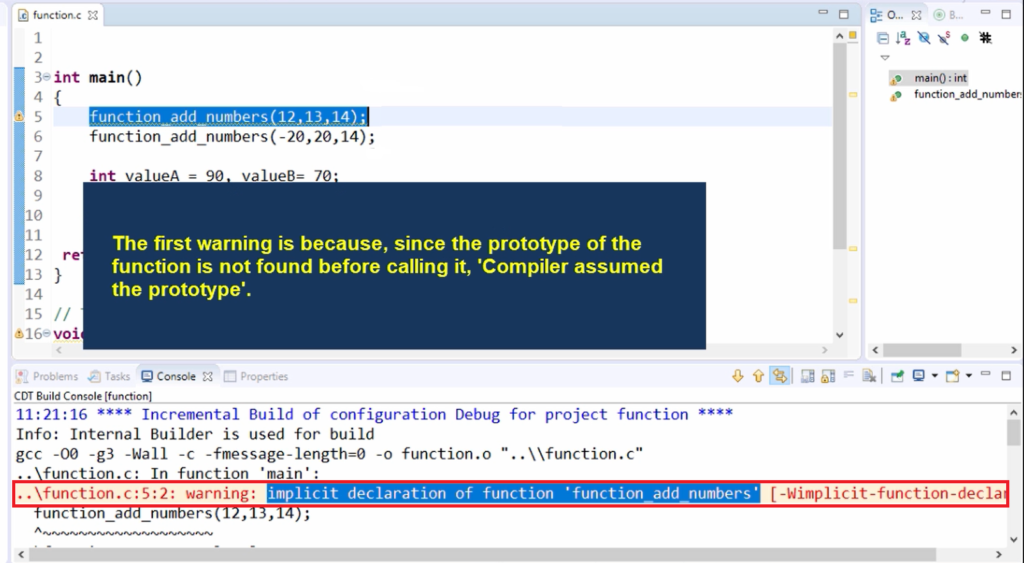
If you observe the error here(Figure 1). It is saying that implicit declaration of function ‘function_add_numbers’.
What does that mean?
Basically, the compiler says that, here(line 5), I encountered a user-defined function usage, but its prototype is not found. That’s why the compiler is assuming the prototype. So, it assumes something else, and after that, the compiler proceeds.
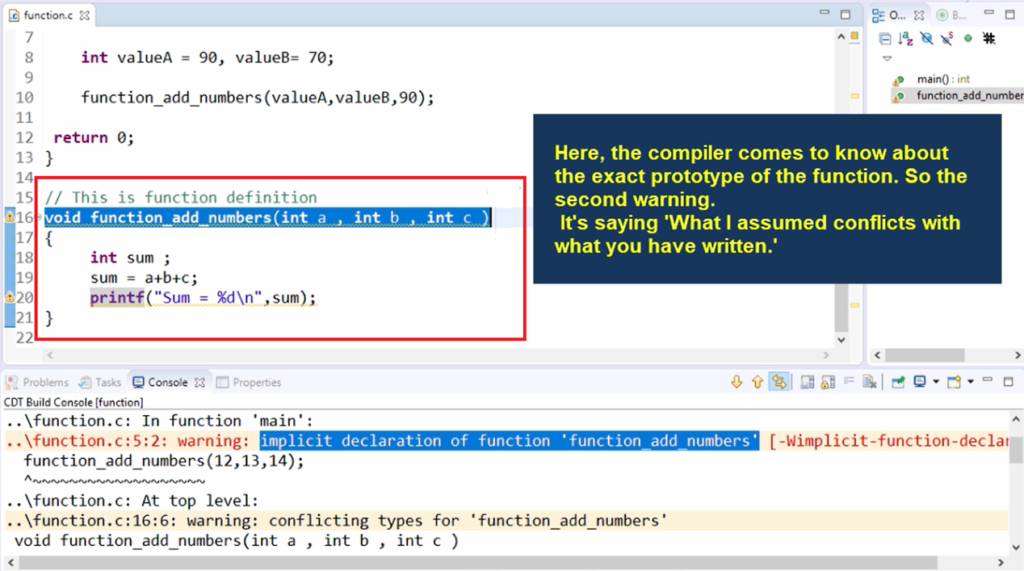
After that, it comes to the real function definition, which is implemented here(as shown in Figure 2).
And here, the compiler comes to know about the actual prototype, and that’s why the second warning is conflicting types for ‘function_add_numbers.’ There is a conflict between what it has assumed here and what it has found here. This you can leave.
After that, the third warning is implicit declaration of function printf. Because we have used printf here, but we didn’t tell the prototype of that. And we do that by using #include <stdio.h>. Because printf is not written by us. So, it is written by standard library code. That’s why we have to give a standard library header file to include the prototype.

After that, we have to mention the prototype of the void function_add_numbers(int a, int b, int c) function before you call that function. That’s why you have to copy that line, go to the global scope and paste that line, and give the semicolon. Now before calling the function, the compiler will encounter the prototype. So, you need not mention int a, int b, int c; you can even mention just int, int, int. Then you compile this program. It gives no warnings.
And after that, since it is a void function, you need not to mention any return value here in this function.
When function_add_numbers(12,13,14) function is called, the value 12 will be copied into int a, 13 will be copied into int b, and 14 will be copied into int c. So, the number of arguments here should match the number of arguments that you pass here. So, otherwise, it will be a problem.
For example, if I pass two arguments like 12 and 13, then the compiler will not let you to proceed, so that’s an error. It is saying that, “too few arguments to function ‘function_add_numbers’”, as shown in Figure 4.
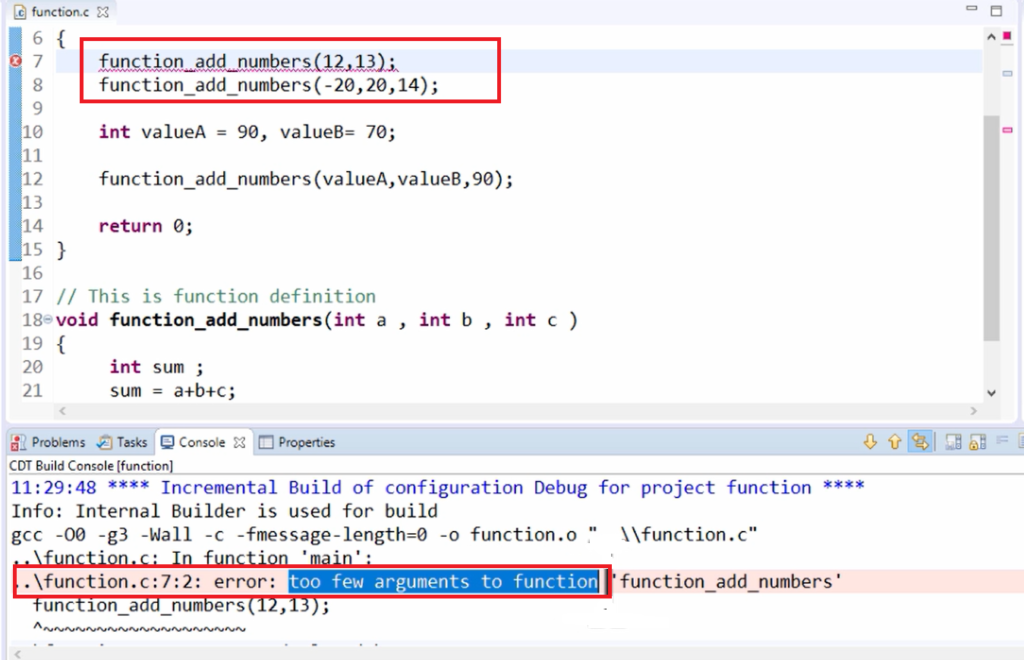
So, give the three arguments. The Code shown below.
#include <stdio.h> int function_add_numbers(int , int , int ); int main() { function_add_numbers(12,13,0); function_add_numbers(-20,20,14); int valueA = 90, valueB = 70; function_add_numbers(valueA, valueB, 90); return 0; }
Compile it. And then execute the program. It is working and shows the output(Figure 5).
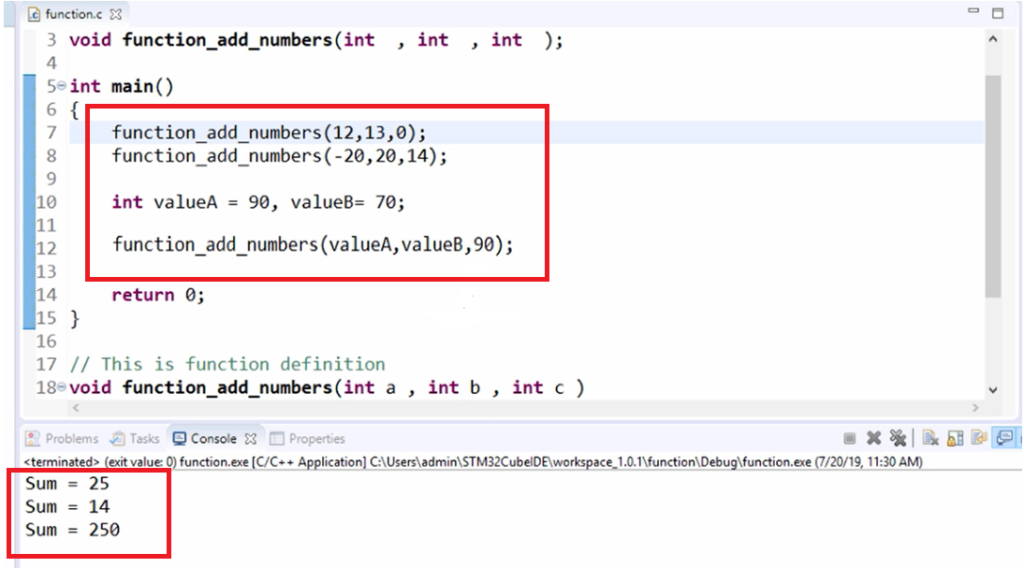
I hope you understood about writing a simple function definition, and in the following article, let’s see how to return a value from a function.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1