What is Null Pointer in C?
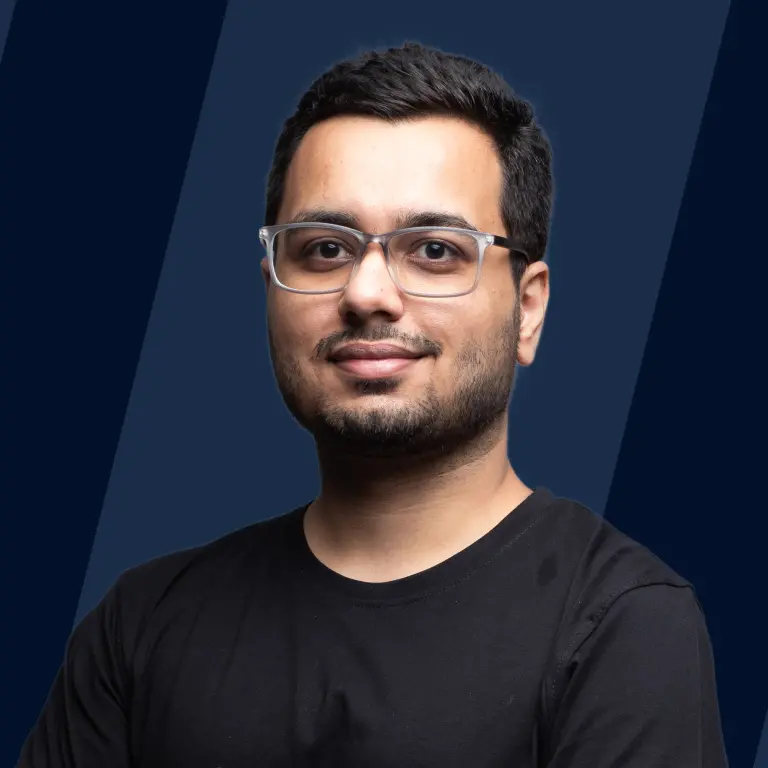
In the C programming language, a null pointer is a pointer that does not point to any memory location and hence does not hold the address of any variables. It just stores the segment's base address. That is, the null pointer in C holds the value Null, but the type of the pointer is void. A null pointer is a special reserved value declared in a header file called stddef. Null indicates that the pointer is pointing to the first memory location. meaning that 0th location.
At a high level, NULL is a null pointer that is implemented in C for a multitude of scenarios.
- To initialize a pointer variable when it hasn't been assigned a proper memory address yet.
- Before accessing any pointer variable, check for a null pointer. We can handle errors in pointer-related code this way, for example, only dereference a pointer variable if it is not NULL.
- When we don't want to pass a valid memory address to a function argument, we can pass a null pointer.
generally, we can say that a null pointer is a pointer that does not point to any object.
Syntax
The syntax of a null pointer can be defined in two ways.
Or
Applications of Null Pointer in C
A Null pointer can be used in the following ways:
- When the pointer variable does not point to a valid memory address, it is used to initialize it.
- Before dereferencing pointers, it is utilized to execute error handling.
- When we don't want to pass the memory location directly, we pass it as a function argument, which is then utilized to return from a function.
Example
Example of Null Pointer in C
We can assign 0 directly to the pointer so that it will not point to any of the memory locations.
Output
Explanation: In the above example, we initialized three pointers as *ptr1, *ptr2, and *ptr3, and we assigned a value to the num variable in *ptr1 and compared it by 0 because *ptr1 is not equal to null, therefore it would output the result as NOT NULL, and we did not assign any value to *ptr2 and As a result, the output will be printed as NOT NULL. We assigned value 0 to *ptr3, which is equal to null, hence the output will be NULL.
Some More Examples
Example 1. This Example Illustrates Inappropriate Use of the NULL Constant for Overloaded Functions
Assume you want func(int* i) to be called from the main function. Because the constant NULL is equal to the integer 0, the main function calls func(int i) instead of func(int* i) Only when func(int i) do not not exist is constant 0 implicitly turned to (void*)0.
Example 2. This Example Illustrates How nullptr is Used in Overloading Functions
Example 3. The Following Expressions Illustrate the Correct and Incorrect Use of the nullptr Constant
Example 4. This Example Illustrates that a non-type Template Parameter or Argument Can Have the std::nullptr_t Type
Example 5. This Example Illustrates How to Use nullptr in Exception Handling
How Does Null Pointer Work in C?
In C, a null pointer is a variable that has no valid address and is allocated to zero or NULL. Normally, the null pointer does not point to anything. NULL is a macro constant defined in several header files in the C programming language, including stdio.h, alloc.h, mem.h, stddef.h, and stdlib.h. Also, take note that NULL should only be used when working with pointers.
Best Practices for NULL Pointer Usage
To avoid programming errors, use a NULL pointer as a best practice.
- Before using a pointer, make it a practice to assign it a value. Don't utilize the pointer before it's been initialized.
- If you don't have a valid memory location to store in a pointer variable, set it to NULL instead.
- Check for a NULL value before utilizing a pointer in any of your function code.
What are the Uses of NULL Pointer in C?
Avoid Crashing a Program
Our program may crash if we pass any garbage value in our code or to a certain method. We can avoid this by using a NULL pointer.
To avoid crasing in a program we can write the code like this:
Output
Explanation: We define function fun1() in the following code and pass a pointer ptrvarA to it. When the function fun1() is called, it checks whether the passed pointer is a null pointer or not. So we must verify if the pointer's passed value is null or not, because if it is not set to any value, it will take the garbage value and terminate your program, resulting in the program crashing.
While Freeing (de-allocating) Memory
Assume you have a pointer pointing to a memory location where data is stored. If you don't need the data any longer, you should remove it and free up some memory.
However, even after the data has been freed, the pointer still points to the same memory location. A dangling pointer is a name given to this type of pointer. Set the pointer to NULL to get rid of the dangling pointer.
NULL Pointer Uses in Linked-List
The Data and Link fields are contained in every node. The data field holds the actual data that we intend to save in the linked list. The link connects each node to the other nodes. The head of the Linked List has a pointer link to the first node. Because it does not point to another node in the Linked List, the last node-link has a null pointer. This null variable is updated with the pointer of the new node when we add a new node at the end of the Linked List.
In Linked List, a NULL pointer is also significant. We know that in a Linked List, we use a pointer to connect one node to its successor node.
Because there is no successor node for the last node, the link of the last node must be set to NULL.
Output
Examples to Implement Null Pointer in C
Example - 1:
Output
Example - 2:
Output:
Example - 3:
Output:
Example - 4:
Output:
Null Pointer Use Cases in C Language
When we Do not Assign any Memory Address to the Pointer Variable
In the example below, we declared the pointer variable *ptr, but it has no variable address. Dereferencing the uninitialized pointer variable will result in a compile-time error because it does not point to any variable. The C program that follows creates a variety of spectacular results before ultimately crashing. As an outcome, we can deduce that a program can crash if an uninitialized pointer is left in it.
How to Avoid the Above Problem?
We can overcome the above problem in the C programming language by utilizing a Null pointer. A null pointer refers to the 0th memory location, which is a shared memory area that can't be dereferenced. In the example below, We create a pointer *ptr and assign it the value NULL to indicate that it doesn't point to any variables. We add a condition after creating a pointer variable that checks if the value of the pointer is null.
Output:
When We Use the malloc() Function
The built-in malloc() method is used to allocate memory in the example below. If the malloc() function fails to allocate memory, the result is a NULL pointer. As an outcome, a condition to check whether the value of a pointer is null or not must be included; if the value of a pointer is not null, memory must be allocated.
Output:
It's usually good programming practice to set a Null value to the pointer when we don't know the actual memory address.
Difference between NULL and Void Pointer
Void Pointer | NULL Pointer |
---|---|
In C, the void is a data type. | The value that is assigned to the pointer is NULL. |
A void pointer is a pointer that has no data type associated with it. | The term "null pointer" refers to a pointer that does not point to anything. |
A general purpose pointer is another name for it. It can store any data type's address. | Set a pointer's address to null if no address has been allocated to it. |
Learn more
Learn more about Pointers in C.
Conclusion
- When we don't know the actual address of memory, it's always useful to set a Null value to the pointer.
- A Null pointer is a variable in the C programming language that has a value of zero or has an address that points to nothing.
- In C, we use the keyword NULL to make a variable a null pointer, which is a predefined macro.